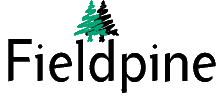
Webhooks
Connect to FD1
FD1 Authentication
Access to FD1 protocol is restricted. To read or write your app must either:
- Connect to a websocket server and provide session keys using the endpoint fd1.session.login Some servers may not require this, or some data may be available publicly
- Obtain a connection ticket, connecting to the websocket server with the connection ticket in the socket address. You may still need to send session keys depending on what you are connecting too
When using Websockets, your authorisaton details are sent using a JSON packet as the first exchange
GET/POST HTTP Requests
When using fetch() style calls and individual requests, your API Key is sent in the header "x-api-key"
When using GET/POST requests, your authorisaton details are sent in HTTP headers
Web Socket Requests
The HTTP authorisation header, which is commonly used for authentication may be required in addition to the api-key. Many sites require authorisation headers to access the server
Your app or Web Page | ⇒ |
Connects to WebSocket URL
https://your-server.com/fd1/open_websocket |
⇒ | WebSocket Open | ⇒ |
Sends fd1.session.login packet to authenticate user. |
⇒ | WebSocket Authorised |
Or ⇒ |
Connects to WebSocket URL
https://your-server.com/fd1/open_websocket?ticket=abc123 |
|||||||
Web Socket is open, but
may not be authorised |
Public Facing Web Sites
If you are wanting to consume FD1 for internet facing applications, such as to the general public without authorisation then we suggest
- Implement your calls to FD1 on your web server, not directly from client browsers
- Consider using one of the proxy options, such that clients connect to the proxy rather than directly to an FD1 server.
Example - Simple
- Create your webpage (HTML)
- Open your websocket wss://your-server.com/fd1/open_websocket
- The server will reply with a hello JSON packet, but this will typically be empty until you authenticate
-
Send your authentication packet
{ a: "fd1.session.login", v: { apikey: "your-api-key", ... optional additional arguments } }
- Server will reply with acknowledgement or error
- You can now call any endpoint you are authorised too
Example - Simple, with GeoLocation
- Create your webpage (HTML)
- Using standard Javascript request users Geo Position
var sGeo = null; var ServerSocket = null; // We ask for Geo co-ords if we can, as these help position what devices can be used if (navigator.geolocation) navigator.geolocation.getCurrentPosition(function (pos) { sGeo = pos.coords; if ((ServerSocket != null) && (ServerSocket.readyState == 1)) { // Geo details have arrived, socket is already connected, so send using session set option let req = { a: "fd1.session.set_option", v: { geo: { latitude: sGeo.latitude, longitude: sGeo.longitude, accuracy: sGeo.accuracy, altitude: sGeo.altitude, altitudeAccuracy: sGeo.altitudeAccuracy } } } ServerSocket.send(JSON.stringify(req)); } });
- Open your websocket wss://your-server.com/fd1/open_websocket
ServerSocket = new WebSocket("wss://your-server.com/fd1/open_websocket");
- The server will reply with a hello JSON packet, but this will typically be empty until you authenticate
-
Send your authentication packet. This is likely to be running before Geo Position returns
let req = { a: "fd1.session.login", v: { apikey: "your-api-key" } } // If we already have Geo details, send it now if (sGeo) { req.v.geo = { latitude: sGeo.latitude, longitude: sGeo.longitude, accuracy: sGeo.accuracy, altitude: sGeo.altitude, altitudeAccuracy: sGeo.altitudeAccuracy } } ServerSocket.send(JSON.stringify(req));
- Server will reply with acknowledgement or error
- You can now call any endpoint you are authorised too