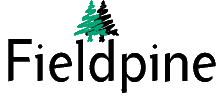
Primitives
Primitives are low level english like commands that can be used to control and influence the Point of Sale and stock management components. Primitives are similar to API calls but can be generated from a variety of sources. Primitives are frequently used when creating user interfaces as they can be easier to learn and use than dealing with many of the lower level API calls.
A quick example - the following primitives create a sale, add an item for $2 and complete the sale with cash payment. This might be a typical quick-sell button.
:sale id new :sale add pid 10 price 2.00 :sale pay type cash amount 2.00
How to send primitives to Fieldpine
From a Webpage?
Call the function FieldpinePrimitive directly from Javascript. (Tip, if you are looking to create your own Front Counter user interface with Primitives, see HTML User Interfaces)
FieldpinePrimitive(":sale add plu ab23");or directly as a onclick handler
<input type="button" value="Newspaper" onclick="FieldpinePrimitive(':sale add pid 100 qty 1')" />
They are quite easy to work with in complex functions where you can merge arrays using join to send multiple commands in one hit
function SellProductAtHalfPrice(theSaleId, thePid, extraArgs) {
var AllCommands = new Array;
AllCommands.push(":sale id " + theSaleId);
var t = new Array
t.push(":sale add pid");
t.push(thePid);
if (extraArgs != null) t.push(extraArgs);
AllCommands.push(t.join(" ")); // Space seperated
AllCommands.push(":sale alter line sequence active discount 50%");
/*
AllCommands is now an array with several elements, if we join with a new line character the resulting string sent to Fieldpine might be
:sale id 1234
:sale add pid 7788 serial(ABC456J)
:sale alter line sequence active discount 50%
*/
FieldpinePrimitive(AllCommands.join("\n"));
/* Or if you prefer simply pass the Array,
although this is slightly less optimal as the called function needs to decode the array
FieldpinePrimitives(AllCommands)
*/
}
From a Command Line?
From Excel?
By Email?
Direct HTTP?
TBS
Some Examples
:sale id new :sale add pid x qty 2 :sale phase void :sale delete line sequence 3 :sale pay type cash amount 2.00
Syntax
Primitive commands are line delimited. This means you should put one command per line and seperate multiple lines with return characters (technically any combination of CR or LF)
You can send multiple primitive command lines in one go.
The general syntax is
:area [state-variables] command [key-values]
Area commands always start with a colon and are used to dispatch internally to the correct processing unit. Some examples of areas are :sale :stock
Key-Values take the form "keyname value", which can be supplied using any of several formats. These formats are identical except for the () form which is required if the value needs to contain whitepsace
keyname value keyname(value) keyname=valueIf you need to send an explicit blank/empty value, use
keyname()
Keyname may include an optional encoding qualifier which can be useful if the data potentially includes characters required by this protocol (newline, brackets, spaces).
Encoding | Example | Example effect |
base64url or b64
Encode the value using "safe Base64" as defined in RFC4648. If encoding from Javascript use the following btoa(unescape(encodeURIComponent( VALUE ))) |
comments/base64url(YWJj) comments/b64(YWJj) | Sets "comments" field to "abc" (decoded YWJj) |
base64
Encode the value using standard Base64 (with + and / characters used). |
orderno/base64url(Zm9v) | Sets "OrderNo" field to "foo" (decoded Zm9v) |
If you need to send key-values that potentially include characters used by the primitives protocol you can use the "transfer" state variable. This allows you to encode the value in a variety of formats, and is especially useful when sending user entered free form text
Parameters can be used with the format ?name. Parameters are not yet supported and this is reserved as a placeholder. For example
:sale add pid ?myvariable
Syntax Checking
It is possible to send commands into the Primitives() functions and have it syntax check only
Responses and Errors
Each Primitive call returns the following standard header
{ "data": { "PrimitiveRun": [ { "Cmd": "....", // Command issued "ElapsedTicks": 15, Per command results } ] }}
The "per command results" is zero or more fields/objects depending on the command executed. Almost all primitives will return call specific data in a "PosData" object
{ "data": { "PrimitiveRun":[ { "Cmd":":getdata activeuistate", // Command issued "DataName":"activeuistate", "ElapsedTicks":15, "PosData":[ // Per command result { "ChangeCounter":0, "ChangeCounterDtHttp":"Sat, 14 Aug 2021 09:24:44 GMT", "SaleCount":1, "ActiveSaleId":830623746, "PaymentType":[ {"TypeStr":"Cash","Status":0}, {"TypeStr":"EFTPOS","Status":0} ], "Flags":[{ "HaveAccount":1, "HaveCustomer":1 }], "References":[{ "StoreServer":[{ "Address":"127.0.0.1:8310" }] }] }] }] }}
If an error is encountered, then an "Error" object is returned