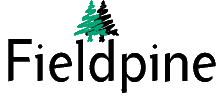
Eftpos Payment Examples
PRELIMINARY. Subject to change without warning
The /Device/Eftpos API allows you to request Eftpos payment directly from your application or web page. This requires an approved pinpad and possible eftpos vendor certifications in some cases.
Overview
- Call /Lane/{Key} to retrieve details of whether an eftpos terminal is available for use.
- Call /Device/Eftpos/RequestPayment to start the payment transaction
- Call /Device/EFtpos/PaymentStatus to check the ongoing status of the payment
Checking if Eftpos available
Every lane within Fieldpine is allocated a unique id, typically called the Srcuid. This number identifies a specific device, and from there details about the device such as which store it operates in and available devices. If you do not know your Srcuid/Unique Id you will need to find this first (@@ TBD. Show how)
The API /Lane/{Srcuid} returns details about your lane
var MyRmSystem = "1_2_3_4"; var MySrcuid = 503; var MyApiKey = "abcbcbcbcbcbcbcbc"; var req = null; if(window.XMLHttpRequest) req = new XMLHttpRequest(); else if (window.ActiveXObject) req = new ActiveXObject("Microsoft.XMLHTTP"); req.open( "GET", "/OpenApi_" + MyRmSystem + "/Lane" + MySrcuid, true); if (window.ActiveXObject) req.setRequestHeader("If-Modified-Since", "Sat, 1 Jan 2000 00:00:00 GMT"); req.setRequestHeader("X-Api-Key", MyApiKey); req.withCredentials = true; req.onreadystatechange = function() { if(req.readyState === 4) { if(req.status === 200) { var reply = JSON.parse(req.responseText); var EftCount = eLink_ExtractNum(reply, "data.LaneRegister[0].EftposCount", 0); if (EftCount > 0) { // Yes, we have at least one eftpos terminal } } } }; req.send();
Requesting Payment
// Create the payment request object var payment = new Object; payment.Amount = 5.99; // Send to server var MyRmSystem = "1_2_3_4"; var MySrcuid = 503; var MyApiKey = "abcbcbcbcbcbcbcbc"; var req = null; if(window.XMLHttpRequest) req = new XMLHttpRequest(); else if (window.ActiveXObject) req = new ActiveXObject("Microsoft.XMLHTTP"); req.open( "POST", "/OpenApi_" + MyRmSystem + "/Device/Eftpos/RequestPayment", true); if (window.ActiveXObject) req.setRequestHeader("If-Modified-Since", "Sat, 1 Jan 2000 00:00:00 GMT"); req.setRequestHeader("X-Api-Key", MyApiKey); req.withCredentials = true; req.setRequestHeader("Content-Type", "application/json"); req.onreadystatechange = function() { if(req.readyState === 4) { if(req.status === 200) { var reply = JSON.parse(req.responseText); DisplayEftposReply(reply); } } }; req.send(payment);
Once the payment has been initiated the pinpad should kick into life and request card swipe. However, you are required to display various messages and controls to on your screen to the person starting the payment process.
Eftpos Terminal Types
There are a couple of ways to connect to eftpos termainals and ways these can be configured. How the pinpad is connected may influence what you can and must do.
Aux App to Pos
Your Web App
EftposPaymentStatus |
« Request Payment » |
PosGreen on Windows PC
Controls Payment |
« interface to » | Eftpos Pinpad |
With this mode of operation, the pinpad is connected to Fieldpine PosGreen and your web app causes PosGreen to start and manage the eftpos process. This means that all prompts and controls primarily appear on the PosGreen lane. Your web app might receive status messages, but to fully control the transaction the operator may need to use the PosGreen application.
This mode allows you to use a pinpad directly connected to your Pos on a mobile device within your store. Essentially the web app triggers the Point of Sale to process an Eftpos payment
Network Pinpads
Your Web App
Controls Payment EftposPaymentStatus |
« Request Payment » | Server | « interface to » | Eftpos Pinpad |
With this mode of operation, the pinpad exists somewhere in the network and your payment request goes directly to the pinpad.
Processing In Progress Transactions
While an Eftpos transaction is in progress you will receive EftposPaymentStatus objects. This both inform you of the current status and any actions you need to do
A key field in the response is "ControlsPayment" field, which is a coded number
- 0. The payment is being controlled elsewhere and you are only able to receive status messages
- 1. You are controlling the payment process and must display messages
- 2. The payment is being controlled elswhere but you may have some ability to influence this remotely
The pseudo logic for payments is
- Create a display area to show transaction progress. This should probably be a modal style window. You may like to display this even if you are not controlling the payment
- Process commands inside EftposPaymentStatus Objects
- If the transaction is not complete and less than 10 minutes have elapsed, poll for additional EftposPaymentStatus objects and repeat the above steps as required